지금까지 iOS LiDAR를 이용하여 거리를 측정하고 상단의 이미지를 추출하는 것을 진행하였습니다. 이번에는 거리에 따라 notification 즉 알림을 보내는 기능을 추가하는 방법에 대해 글을 쓰고자 합니다.
unity에서 notification을 사용하기 위해서는 우선
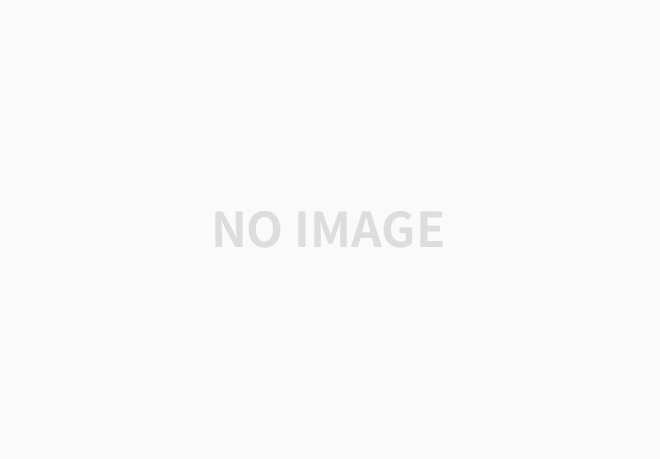
위 사진과 같이 Mobile Notification 패키지를 import 해줘야합니다.
해당 패키지를 다운하면 이제 android와 iOS에서 notification을 사용할 수 있습니다.
이제 script를 이용하여 사용을 해주면 되는데 기본적인 내용은 이곳에서 볼 수 있으며 유튜브 강의는 여기를 참고하시면 될 것 같습니다.
먼저 notification을 사용하기 위한 기본적은 script를 작성할 필요가 있는데 해당 코드는 아래와 같습니다.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using Unity.Notifications.iOS;
public class iosNotificationController : MonoBehaviour
{
//notification 사용하기 위함
public IEnumerator<string> RequestAuthorization()
{
using (var req = new AuthorizationRequest(AuthorizationOption.Alert | AuthorizationOption.Badge, true))
{
while (!req.IsFinished)
{
yield return null;
}
}
}
public void SendNotification(string title, string body, string subtitle, int fireTimeInSeconds)
{
var timeTrigger = new iOSNotificationTimeIntervalTrigger()
{
TimeInterval = new System.TimeSpan(0, 0, fireTimeInSeconds),
Repeats = false
};
var notification = new iOSNotification()
{
Identifier = "hello_world_notification",
Title = title,
Body = body,
Subtitle = subtitle,
ShowInForeground = true,
ForegroundPresentationOption = (PresentationOption.Alert | PresentationOption.Sound),
CategoryIdentifier = "default_category",
ThreadIdentifier = "thread1",
Trigger = timeTrigger
};
iOSNotificationCenter.ScheduleNotification(notification);
}
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
}
}
위 코드에서
var timeTrigger = new iOSNotificationTimeIntervalTrigger()
{
TimeInterval = new System.TimeSpan(0, 0, fireTimeInSeconds),
Repeats = false
};
이 부분이 notification의 시간 간격을 설정하는 부분이라 1초보다 더 자주 notification을 주기위해 int로 설정되어 있는 TimeInterval을 double로 변경하니 오류가 났습니다.
그래서 아쉽게 현재 가장 빠른 주기인 1초를 기준으로 코드를 진행하였습니다. ㅠㅠ (혹시 변경법을 아시는 분은 알려주시면 감사하겠습니다!!)
이제 기본적은 코드는 작성하였으니 사용하기 위한 코드가 필요하다. 사용하기 위한 코드는
이전에 글에서 만든 코드에
// notification 불러오기
[SerializeField]
private iosNotificationController iosNotificationController;
위 코드로 script를 가져온 후 void Start() 부분에 notification 사용 승인을 질문하는
StartCoroutine(iosNotificationController.RequestAuthorization());
코드를 추가하였습니다.
이전 글에서 작성한 것을 사용하여 1.3m 보다 가까워졌을 때 빨간 표기와 함께 notification도 동작하길 바라며 void Update()에
// first는 제목부분, warning은 body부분, subtitle은 말그대로, 1은 TimeInterval 시간이다.
iosNotificationController.SendNotification("first", "warning", "subtitle", 1);
을 넣으니 처음에는 동작을 하지 않았습니다.
알고보니 Unity의 Update의 속도가 TimeInterval인 1초 보다 빨라 notification문구가 나오지 않았고, 앱을 종료하면 그제서야 나오는 상황이 나타난 것.....
(이 이유를 몰라서 하루를 썼다...)
문제의 원인을 알게되어 해당 문제를 해결하기 위해 Update가 아닌 FixedUpdate()를 이용하였습니다.
FixedUpdate는 매 프레임마다 실행되는 Update와 달리 원하는 주기를 설정하여 해당주기에 따라 Update시킬 수 있기에 원하는 주기를 1초 보다 크게 한다면 동작에 문제가 없을 것이라 판단하였습니다.
FixedUpdate의 주기를 변경하기 위해서는 project setting의 player Setting에 들어가야 합니다!!
그 후
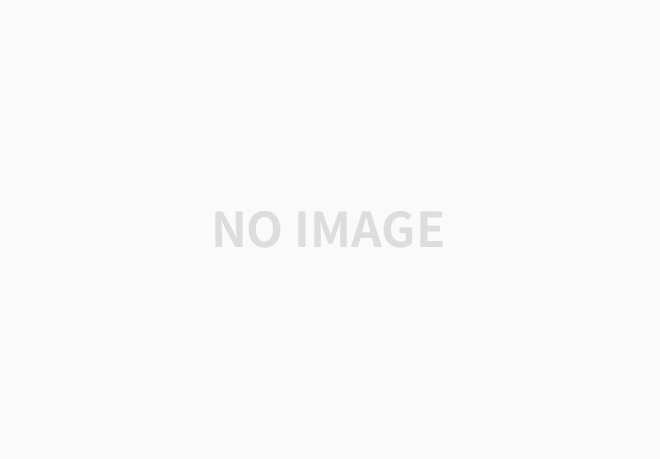
사진과 같이 Time의 가장 상단인 Fixed Timestep을 변경하면 원하는 주기를 설정할 수 있습니다.
위 사진과 같이 작성하면 주기는 1.1초가 되는거죠~
주기를 설정한 후 iosNotificationController를 FixedUpdate에 사용하기 위해
// notification을 실행시키기 위한 trigger
static int trigger;
static을 이용하여 trigger라는 전역변수를 만들고 1.3m보다 가까워졌을 때
if (hitDis < 1.3f)
{
trigger = 0;
color[i].material.color = Color.red;
Debug.Log("거리가 가까워짐");
trigger = 1;
}
다음과 같이 trigger가 1이 되도록 했습니다.
그리고
private void FixedUpdate()
{
if(trigger == 1)
{
iosNotificationController.SendNotification("first", "warning", "subtitle", 1);
trigger = 0;
}
}
다음과 같이 동작 후 초기화를 해주니 원하는 동작이 실행되었습니다!!
원래는 실시간성이 가장 중요하여 1초 보다 빠른 반응이 필요하지만 아직 해당 부분은 좀 더 공부가 필요해 보이네요...ㅠ

실행결과 위 사진처럼 아주 잘 동작하는 것을 볼 수 있습니다!! 영상을 보고싶은신 분은 이곳을 눌러주세요~
To Be Continued...
'LiDAR' 카테고리의 다른 글
iOS 가로 세로 모드 설정 (0) | 2024.03.03 |
---|---|
Flutter + Unity AR (0) | 2024.02.27 |
Unity AR iOS LiDAR 활용 (0) | 2024.02.26 |
Unity AR 메모리 누수 현상 잡기 (0) | 2024.02.25 |
Unity AR 딥러닝 사용하기 (2) | 2024.02.25 |